Nearest-neighbour indexing using xoak#
This notebook experiments subsetting datasets using TLONG, TLAT, ULONG, ULAT
by making use of the xoak package
import matplotlib as mpl
import numpy as np
import xarray as xr
import xoak
import pop_tools
---------------------------------------------------------------------------
ModuleNotFoundError Traceback (most recent call last)
Cell In[1], line 4
2 import numpy as np
3 import xarray as xr
----> 4 import xoak
6 import pop_tools
ModuleNotFoundError: No module named 'xoak'
# open sample data
filepath = pop_tools.DATASETS.fetch('Pac_POP0.1_JRA_IAF_1993-12-6-test.nc')
ds = xr.open_dataset(filepath)
# get DZU and DZT, needed for operations later on
filepath_g = pop_tools.DATASETS.fetch('Pac_grid_pbc_1301x305x62.tx01_62l.2013-07-13.nc')
ds_g = xr.open_dataset(filepath_g)
ds.update(ds_g)
<xarray.Dataset> Dimensions: (bnds: 2, nlat: 305, nlon: 1301, time: 1, z_t: 62, z_t_150m: 15, z_w: 62, z_w_bot: 62, z_w_top: 62) Coordinates: * time (time) object 0036-12-07 00:00:00 TLONG (nlat, nlon) float64 ... ULAT (nlat, nlon) float64 ... * z_t (z_t) float32 500.0 1.5e+03 ... 5.625e+05 5.875e+05 TLAT (nlat, nlon) float64 ... ULONG (nlat, nlon) float64 ... * z_t_150m (z_t_150m) float32 500.0 1.5e+03 ... 1.35e+04 1.45e+04 * z_w (z_w) float32 0.0 1e+03 2e+03 ... 5.5e+05 5.75e+05 * z_w_bot (z_w_bot) float32 1e+03 2e+03 3e+03 ... 5.75e+05 6e+05 * z_w_top (z_w_top) float32 0.0 1e+03 2e+03 ... 5.5e+05 5.75e+05 Dimensions without coordinates: bnds, nlat, nlon Data variables: (12/32) time_bnds (time, bnds) object 0036-12-02 00:00:00 0036-12-07 00:... UAREA (nlat, nlon) float64 ... TAREA (nlat, nlon) float64 ... DXU (nlat, nlon) float64 ... DYU (nlat, nlon) float64 ... DXT (nlat, nlon) float64 ... ... ... dzw (z_w) float32 500.0 1e+03 1e+03 ... 2.5e+04 2.5e+04 grav float64 980.6 nsurface_t float64 5.413e+06 nsurface_u float64 5.372e+06 omega float64 7.292e-05 radius float64 6.371e+08 Attributes: (12/13) CDI: Climate Data Interface version 1.9.2 (http://mpimet.mp... history: Tue Mar 24 11:51:32 2020: cdo selvar,UVEL,VVEL,DXT,DXU... source: CCSM POP2, the CCSM Ocean Component Conventions: CF-1.0; http://www.cgd.ucar.edu/cms/eaton/netcdf/CF-cu... title: g.e20.G.TL319_t13.control.001_hfreq time_period_freq: day_5 ... ... contents: Diagnostic and Prognostic Variables revision: $Id: tavg.F90 89091 2018-04-30 15:58:32Z altuntas@ucar... calendar: All years have exactly 365 days. start_time: This dataset was created on 2018-12-14 at 16:05:58.8 cell_methods: cell_methods = time: mean ==> the variable values are ... CDO: Climate Data Operators version 1.9.2 (http://mpimet.mp...
xarray.Dataset
- bnds: 2
- nlat: 305
- nlon: 1301
- time: 1
- z_t: 62
- z_t_150m: 15
- z_w: 62
- z_w_bot: 62
- z_w_top: 62
- time(time)object0036-12-07 00:00:00
- standard_name :
- time
- long_name :
- time
- bounds :
- time_bnds
- axis :
- T
array([cftime.DatetimeNoLeap(36, 12, 7, 0, 0, 0, 0)], dtype=object)
- TLONG(nlat, nlon)float64...
- long_name :
- array of t-grid longitudes
- units :
- degrees_east
[396805 values with dtype=float64]
- ULAT(nlat, nlon)float64...
- long_name :
- array of u-grid latitudes
- units :
- degrees_north
[396805 values with dtype=float64]
- z_t(z_t)float32500.0 1.5e+03 ... 5.875e+05
- long_name :
- depth from surface to midpoint of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 500.0
- valid_max :
- 587499.06
array([5.000000e+02, 1.500000e+03, 2.500000e+03, 3.500000e+03, 4.500000e+03, 5.500000e+03, 6.500000e+03, 7.500000e+03, 8.500000e+03, 9.500000e+03, 1.050000e+04, 1.150000e+04, 1.250000e+04, 1.350000e+04, 1.450000e+04, 1.550000e+04, 1.650984e+04, 1.754790e+04, 1.862913e+04, 1.976603e+04, 2.097114e+04, 2.225783e+04, 2.364088e+04, 2.513702e+04, 2.676542e+04, 2.854837e+04, 3.051192e+04, 3.268680e+04, 3.510935e+04, 3.782276e+04, 4.087846e+04, 4.433777e+04, 4.827367e+04, 5.277280e+04, 5.793729e+04, 6.388626e+04, 7.075633e+04, 7.870025e+04, 8.788252e+04, 9.847059e+04, 1.106204e+05, 1.244567e+05, 1.400497e+05, 1.573946e+05, 1.764003e+05, 1.968944e+05, 2.186457e+05, 2.413972e+05, 2.649001e+05, 2.889385e+05, 3.133405e+05, 3.379793e+05, 3.627670e+05, 3.876452e+05, 4.125768e+05, 4.375392e+05, 4.625190e+05, 4.875083e+05, 5.125028e+05, 5.375000e+05, 5.624991e+05, 5.874991e+05], dtype=float32)
- TLAT(nlat, nlon)float64...
- long_name :
- array of t-grid latitudes
- units :
- degrees_north
[396805 values with dtype=float64]
- ULONG(nlat, nlon)float64...
- long_name :
- array of u-grid longitudes
- units :
- degrees_east
[396805 values with dtype=float64]
- z_t_150m(z_t_150m)float32500.0 1.5e+03 ... 1.35e+04 1.45e+04
- long_name :
- depth from surface to midpoint of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 500.0
- valid_max :
- 14500.0
array([ 500., 1500., 2500., 3500., 4500., 5500., 6500., 7500., 8500., 9500., 10500., 11500., 12500., 13500., 14500.], dtype=float32)
- z_w(z_w)float320.0 1e+03 ... 5.5e+05 5.75e+05
- long_name :
- depth from surface to top of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 0.0
- valid_max :
- 574999.06
array([ 0. , 1000. , 2000. , 3000. , 4000. , 5000. , 6000. , 7000. , 8000. , 9000. , 10000. , 11000. , 12000. , 13000. , 14000. , 15000. , 16000. , 17019.682, 18076.129, 19182.125, 20349.932, 21592.344, 22923.312, 24358.453, 25915.58 , 27615.26 , 29481.47 , 31542.373, 33831.227, 36387.473, 39258.047, 42498.887, 46176.656, 50370.688, 55174.91 , 60699.668, 67072.86 , 74439.805, 82960.695, 92804.35 , 104136.82 , 117104.016, 131809.36 , 148290.08 , 166499.2 , 186301.44 , 207487.39 , 229803.9 , 252990.4 , 276809.84 , 301067.06 , 325613.84 , 350344.88 , 375189.2 , 400101.16 , 425052.47 , 450026.06 , 475012. , 500004.7 , 525000.94 , 549999.06 , 574999.06 ], dtype=float32)
- z_w_bot(z_w_bot)float321e+03 2e+03 ... 5.75e+05 6e+05
- long_name :
- depth from surface to bottom of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 1000.0
- valid_max :
- 599999.06
array([ 1000. , 2000. , 3000. , 4000. , 5000. , 6000. , 7000. , 8000. , 9000. , 10000. , 11000. , 12000. , 13000. , 14000. , 15000. , 16000. , 17019.682, 18076.129, 19182.125, 20349.932, 21592.344, 22923.312, 24358.453, 25915.58 , 27615.26 , 29481.47 , 31542.373, 33831.227, 36387.473, 39258.047, 42498.887, 46176.656, 50370.688, 55174.91 , 60699.668, 67072.86 , 74439.805, 82960.695, 92804.35 , 104136.82 , 117104.016, 131809.36 , 148290.08 , 166499.2 , 186301.44 , 207487.39 , 229803.9 , 252990.4 , 276809.84 , 301067.06 , 325613.84 , 350344.88 , 375189.2 , 400101.16 , 425052.47 , 450026.06 , 475012. , 500004.7 , 525000.94 , 549999.06 , 574999.06 , 599999.06 ], dtype=float32)
- z_w_top(z_w_top)float320.0 1e+03 ... 5.5e+05 5.75e+05
- long_name :
- depth from surface to top of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 0.0
- valid_max :
- 574999.06
array([ 0. , 1000. , 2000. , 3000. , 4000. , 5000. , 6000. , 7000. , 8000. , 9000. , 10000. , 11000. , 12000. , 13000. , 14000. , 15000. , 16000. , 17019.682, 18076.129, 19182.125, 20349.932, 21592.344, 22923.312, 24358.453, 25915.58 , 27615.26 , 29481.47 , 31542.373, 33831.227, 36387.473, 39258.047, 42498.887, 46176.656, 50370.688, 55174.91 , 60699.668, 67072.86 , 74439.805, 82960.695, 92804.35 , 104136.82 , 117104.016, 131809.36 , 148290.08 , 166499.2 , 186301.44 , 207487.39 , 229803.9 , 252990.4 , 276809.84 , 301067.06 , 325613.84 , 350344.88 , 375189.2 , 400101.16 , 425052.47 , 450026.06 , 475012. , 500004.7 , 525000.94 , 549999.06 , 574999.06 ], dtype=float32)
- time_bnds(time, bnds)object...
array([[cftime.DatetimeNoLeap(36, 12, 2, 0, 0, 0, 0), cftime.DatetimeNoLeap(36, 12, 7, 0, 0, 0, 0)]], dtype=object)
- UAREA(nlat, nlon)float64...
- long_name :
- area of U cells
- units :
- centimeter^2
[396805 values with dtype=float64]
- TAREA(nlat, nlon)float64...
- long_name :
- area of T cells
- units :
- centimeter^2
[396805 values with dtype=float64]
- DXU(nlat, nlon)float64...
- long_name :
- x-spacing centered at U points
- units :
- centimeters
[396805 values with dtype=float64]
- DYU(nlat, nlon)float64...
- long_name :
- y-spacing centered at U points
- units :
- centimeters
[396805 values with dtype=float64]
- DXT(nlat, nlon)float64...
- long_name :
- x-spacing centered at T points
- units :
- centimeters
[396805 values with dtype=float64]
- DYT(nlat, nlon)float64...
- long_name :
- y-spacing centered at T points
- units :
- centimeters
[396805 values with dtype=float64]
- UVEL(time, z_t, nlat, nlon)float32...
- long_name :
- Velocity in grid-x direction
- units :
- centimeter/s
- grid_loc :
- 3221
- cell_methods :
- time: mean
[24601910 values with dtype=float32]
- VVEL(time, z_t, nlat, nlon)float32...
- long_name :
- Velocity in grid-y direction
- units :
- centimeter/s
- grid_loc :
- 3221
- cell_methods :
- time: mean
[24601910 values with dtype=float32]
- TEMP(time, z_t, nlat, nlon)float32...
- long_name :
- Potential Temperature
- units :
- degC
- grid_loc :
- 3111
- cell_methods :
- time: mean
[24601910 values with dtype=float32]
- ANGLE(nlat, nlon)float64...
- long_name :
- angle grid makes with latitude line
- units :
- radians
[396805 values with dtype=float64]
- ANGLET(nlat, nlon)float64...
- long_name :
- angle grid makes with latitude line on T grid
- units :
- radians
[396805 values with dtype=float64]
- DZBC(nlat, nlon)float32...
[396805 values with dtype=float32]
- DZT(z_t, nlat, nlon)float32...
- longname :
- T-grid cell thickness
- units :
- cm
[24601910 values with dtype=float32]
- DZU(z_t, nlat, nlon)float32...
- longname :
- U-grid cell thickness
- units :
- cm
[24601910 values with dtype=float32]
- HT(nlat, nlon)float64...
- long_name :
- ocean depth at T points
- units :
- centimeter
[396805 values with dtype=float64]
- HTE(nlat, nlon)float64...
- long_name :
- cell widths on East sides of T cell
- units :
- centimeters
[396805 values with dtype=float64]
- HTN(nlat, nlon)float64...
- long_name :
- cell widths on North sides of T cell
- units :
- centimeters
[396805 values with dtype=float64]
- HU(nlat, nlon)float64...
- long_name :
- ocean depth at U points
- units :
- centimeter
[396805 values with dtype=float64]
- HUS(nlat, nlon)float64...
- long_name :
- cell widths on South sides of U cell
- units :
- centimeters
[396805 values with dtype=float64]
- HUW(nlat, nlon)float64...
- long_name :
- cell widths on West sides of U cell
- units :
- centimeters
[396805 values with dtype=float64]
- KMT(nlat, nlon)float64...
- long_name :
- k Index of Deepest Grid Cell on T Grid
[396805 values with dtype=float64]
- KMU(nlat, nlon)float64...
- long_name :
- k Index of Deepest Grid Cell on U Grid
[396805 values with dtype=float64]
- REGION_MASK(nlat, nlon)float64...
- long_name :
- basin index number (signed integers)
[396805 values with dtype=float64]
- days_in_norm_year()timedelta64[ns]...
- long_name :
- Calendar Length
array(31536000000000000, dtype='timedelta64[ns]')
- dz(z_t)float32...
- long_name :
- thickness of layer k
- units :
- centimeters
array([ 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1019.6808, 1056.4484, 1105.9951, 1167.807 , 1242.4133, 1330.9678, 1435.141 , 1557.1259, 1699.6796, 1866.2124, 2060.9023, 2288.852 , 2556.247 , 2870.575 , 3240.8372, 3677.7725, 4194.031 , 4804.2236, 5524.7544, 6373.192 , 7366.945 , 8520.893 , 9843.658 , 11332.466 , 12967.199 , 14705.344 , 16480.709 , 18209.135 , 19802.234 , 21185.957 , 22316.51 , 23186.494 , 23819.45 , 24257.217 , 24546.78 , 24731.014 , 24844.328 , 24911.975 , 24951.291 , 24973.594 , 24985.96 , 24992.674 , 24996.244 , 24998.11 , 25000. , 25000. ], dtype=float32)
- dzw(z_w)float32...
- long_name :
- midpoint of k to midpoint of k+1
- units :
- centimeters
array([ 500. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1000. , 1009.8404, 1038.0646, 1081.2218, 1136.901 , 1205.1101, 1286.6906, 1383.0544, 1496.1334, 1628.4027, 1782.946 , 1963.5574, 2174.8772, 2422.5496, 2713.4111, 3055.706 , 3459.305 , 3935.9016, 4499.1274, 5164.489 , 5948.973 , 6870.0684, 7943.9185, 9182.275 , 10588.062 , 12149.832 , 13836.271 , 15593.026 , 17344.922 , 19005.686 , 20494.096 , 21751.234 , 22751.502 , 23502.97 , 24038.332 , 24401.998 , 24638.896 , 24787.672 , 24878.15 , 24931.633 , 24962.443 , 24979.777 , 24989.316 , 24994.459 , 24997.176 , 24999.055 , 25000. ], dtype=float32)
- grav()float64...
- long_name :
- Acceleration Due to Gravity
- units :
- centimeter/s^2
array(980.616)
- nsurface_t()float64...
- long_name :
- Number of Ocean T Points at Surface
array(5413142.)
- nsurface_u()float64...
- long_name :
- Number of Ocean U Points at Surface
array(5371534.)
- omega()float64...
- long_name :
- Earths Angular Velocity
- units :
- 1/second
array(7.292124e-05)
- radius()float64...
- long_name :
- Earths Radius
- units :
- centimeters
array(6.37122e+08)
- CDI :
- Climate Data Interface version 1.9.2 (http://mpimet.mpg.de/cdi)
- history :
- Tue Mar 24 11:51:32 2020: cdo selvar,UVEL,VVEL,DXT,DXU,DYT,DYU,TAREA,UAREA,TEMP Pac_POP0.1_JRA_IAF_1993-12-6.nc Pac_POP0.1_JRA_IAF_1993-12-6-test.nc none
- source :
- CCSM POP2, the CCSM Ocean Component
- Conventions :
- CF-1.0; http://www.cgd.ucar.edu/cms/eaton/netcdf/CF-current.htm
- title :
- g.e20.G.TL319_t13.control.001_hfreq
- time_period_freq :
- day_5
- model_doi_url :
- https://doi.org/10.5065/D67H1H0V
- contents :
- Diagnostic and Prognostic Variables
- revision :
- $Id: tavg.F90 89091 2018-04-30 15:58:32Z altuntas@ucar.edu $
- calendar :
- All years have exactly 365 days.
- start_time :
- This dataset was created on 2018-12-14 at 16:05:58.8
- cell_methods :
- cell_methods = time: mean ==> the variable values are averaged over the time interval between the previous time coordinate and the current one. cell_methods absent ==> the variable values are at the time given by the current time coordinate.
- CDO :
- Climate Data Operators version 1.9.2 (http://mpimet.mpg.de/cdo)
grid, xds = pop_tools.to_xgcm_grid_dataset(ds)
Set the “index”#
This is what allows the indexing magic.
xds.xoak.set_index(['TLAT', 'TLONG'], 'scipy_kdtree')
Extracting sections#
# have to know that 0.1 is a reasonable choice
lons = xr.Variable("points", np.arange(150, 270, 0.1))
lats = xr.zeros_like(lons)
eqsection = xds.xoak.sel(TLONG=lons, TLAT=lats)
# plot
eqsection.TEMP.sel(z_t=slice(50000)).plot(y="z_t", cmap=mpl.cm.Spectral_r, yincrease=False)
<matplotlib.collections.QuadMesh at 0x7f18c3a888e0>
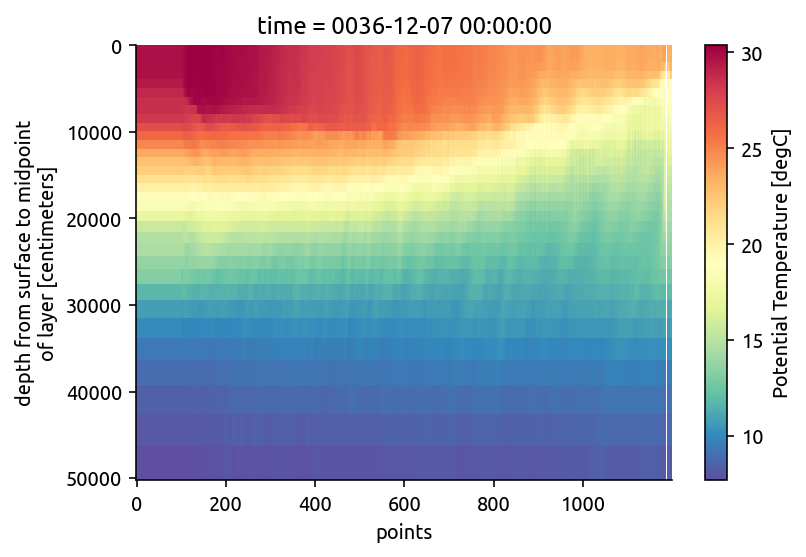
Extracting multiple points#
moorings = xds.xoak.sel(
TLONG=xr.Variable("moor", [360 - 140, 360 - 110]),
TLAT=xr.Variable("moor", [0, 0]),
)
moorings.TEMP.plot(hue="TLONG", y="z_t", yincrease=False)
[<matplotlib.lines.Line2D at 0x7f18c3aa57f0>,
<matplotlib.lines.Line2D at 0x7f18c3aa58e0>]
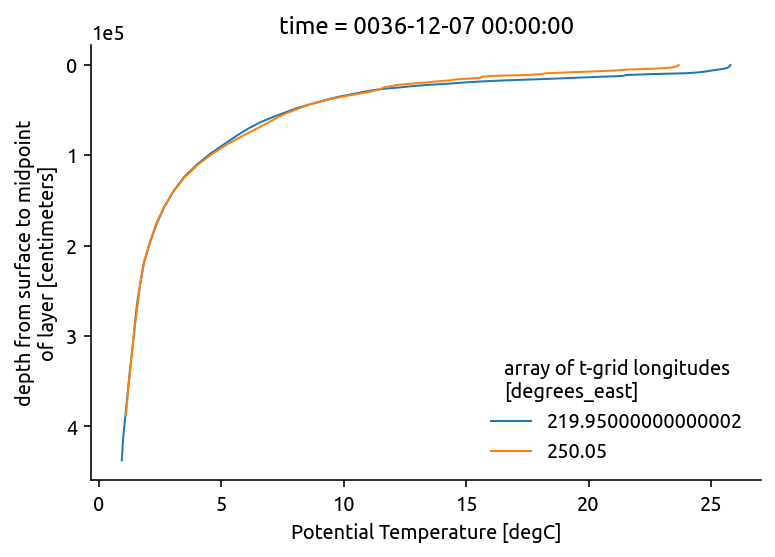
Extracting single points#
xds.xoak.sel(TLONG=360 - 140, TLAT=0).TEMP
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
<ipython-input-63-cc4715aab86e> in <module>
----> 1 xds.xoak.sel(TLONG=360 - 140, TLAT=0).TEMP
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in sel(self, indexers, **indexers_kwargs)
251
252 indexers = either_dict_or_kwargs(indexers, indexers_kwargs, 'xoak.sel')
--> 253 indices = self._query(indexers)
254
255 if not isinstance(indices, np.ndarray):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in _query(self, indexers)
135
136 def _query(self, indexers):
--> 137 X = coords_to_point_array([indexers[c] for c in self._index_coords])
138
139 if isinstance(X, np.ndarray) and isinstance(self._index, XoakIndexWrapper):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in coords_to_point_array(coords)
18
19 """
---> 20 c_chunks = [c.chunks for c in coords]
21
22 if any([chunks is None for chunks in c_chunks]):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in <listcomp>(.0)
18
19 """
---> 20 c_chunks = [c.chunks for c in coords]
21
22 if any([chunks is None for chunks in c_chunks]):
AttributeError: 'int' object has no attribute 'chunks'
xoak expectes “trajectories” to sample along. For a single point we create a 1D variable representing the coordinate location we want: in this case TLONG=220, TLAT=0
. Seems like this could be fixed: xarray-contrib/xoak#37
xds.xoak.sel(
TLONG=xr.Variable("points", [360 - 140]), TLAT=xr.Variable("points", [0])
).TEMP.squeeze("points")
<xarray.DataArray 'TEMP' (time: 1, z_t: 62)> array([[25.794353 , 25.759989 , 25.728874 , 25.674746 , 25.541134 , 25.282745 , 25.026842 , 24.813889 , 24.512192 , 23.9899 , 22.513605 , 21.532747 , 21.387815 , 20.417183 , 19.48939 , 18.584412 , 17.558277 , 16.449156 , 15.601459 , 14.897679 , 14.326382 , 13.539371 , 12.851948 , 12.320872 , 11.637564 , 11.112046 , 10.732501 , 10.340948 , 9.864892 , 9.434968 , 9.031752 , 8.564738 , 8.070933 , 7.620849 , 7.1164446 , 6.5846534 , 6.0993133 , 5.625975 , 5.127931 , 4.5544333 , 4.004363 , 3.477641 , 3.0496979 , 2.6729817 , 2.360019 , 2.0861135 , 1.8473006 , 1.6999204 , 1.5623759 , 1.4673579 , 1.3774252 , 1.2729893 , 1.1820002 , 1.0894325 , 0.99818563, 0.9394488 , nan, nan, nan, nan, nan, nan]], dtype=float32) Coordinates: * time (time) object 0036-12-07 00:00:00 TLONG float64 220.0 * z_t (z_t) float32 500.0 1.5e+03 2.5e+03 ... 5.625e+05 5.875e+05 TLAT float64 -0.05 nlon_t float64 600.5 nlat_t float64 152.5 Attributes: long_name: Potential Temperature units: degC grid_loc: 3111 cell_methods: time: mean
xarray.DataArray
'TEMP'
- time: 1
- z_t: 62
- 25.79 25.76 25.73 25.67 25.54 25.28 25.03 ... nan nan nan nan nan nan
array([[25.794353 , 25.759989 , 25.728874 , 25.674746 , 25.541134 , 25.282745 , 25.026842 , 24.813889 , 24.512192 , 23.9899 , 22.513605 , 21.532747 , 21.387815 , 20.417183 , 19.48939 , 18.584412 , 17.558277 , 16.449156 , 15.601459 , 14.897679 , 14.326382 , 13.539371 , 12.851948 , 12.320872 , 11.637564 , 11.112046 , 10.732501 , 10.340948 , 9.864892 , 9.434968 , 9.031752 , 8.564738 , 8.070933 , 7.620849 , 7.1164446 , 6.5846534 , 6.0993133 , 5.625975 , 5.127931 , 4.5544333 , 4.004363 , 3.477641 , 3.0496979 , 2.6729817 , 2.360019 , 2.0861135 , 1.8473006 , 1.6999204 , 1.5623759 , 1.4673579 , 1.3774252 , 1.2729893 , 1.1820002 , 1.0894325 , 0.99818563, 0.9394488 , nan, nan, nan, nan, nan, nan]], dtype=float32)
- time(time)object0036-12-07 00:00:00
- standard_name :
- time
- long_name :
- time
- bounds :
- time_bnds
- axis :
- T
array([cftime.DatetimeNoLeap(36, 12, 7, 0, 0, 0, 0)], dtype=object)
- TLONG()float64220.0
- long_name :
- array of t-grid longitudes
- units :
- degrees_east
- grid_loc :
- 2110
array(219.95)
- z_t(z_t)float32500.0 1.5e+03 ... 5.875e+05
- long_name :
- depth from surface to midpoint of layer
- units :
- centimeters
- positive :
- down
- valid_min :
- 500.0
- valid_max :
- 587499.06
- axis :
- Z
array([5.000000e+02, 1.500000e+03, 2.500000e+03, 3.500000e+03, 4.500000e+03, 5.500000e+03, 6.500000e+03, 7.500000e+03, 8.500000e+03, 9.500000e+03, 1.050000e+04, 1.150000e+04, 1.250000e+04, 1.350000e+04, 1.450000e+04, 1.550000e+04, 1.650984e+04, 1.754790e+04, 1.862913e+04, 1.976603e+04, 2.097114e+04, 2.225783e+04, 2.364088e+04, 2.513702e+04, 2.676542e+04, 2.854837e+04, 3.051192e+04, 3.268680e+04, 3.510935e+04, 3.782276e+04, 4.087846e+04, 4.433777e+04, 4.827367e+04, 5.277280e+04, 5.793729e+04, 6.388626e+04, 7.075633e+04, 7.870025e+04, 8.788252e+04, 9.847059e+04, 1.106204e+05, 1.244567e+05, 1.400497e+05, 1.573946e+05, 1.764003e+05, 1.968944e+05, 2.186457e+05, 2.413972e+05, 2.649001e+05, 2.889385e+05, 3.133405e+05, 3.379793e+05, 3.627670e+05, 3.876452e+05, 4.125768e+05, 4.375392e+05, 4.625190e+05, 4.875083e+05, 5.125028e+05, 5.375000e+05, 5.624991e+05, 5.874991e+05], dtype=float32)
- TLAT()float64-0.05
- long_name :
- array of t-grid latitudes
- units :
- degrees_north
- grid_loc :
- 2110
array(-0.05000002)
- nlon_t()float64600.5
- axis :
- X
array(600.5)
- nlat_t()float64152.5
- axis :
- Y
array(152.5)
- long_name :
- Potential Temperature
- units :
- degC
- grid_loc :
- 3111
- cell_methods :
- time: mean
Limitations#
[ ] cannot simply index by point
[ ] Can only set one index at a time (so only, TLONG, TLAT or ULONG, ULAT)
[ ] indexes are not propagated so
xds.TEMP.xoak
will only work afterxds.TEMP.set_index
is called
xds.TEMP.xoak.sel(TLONG=220, TLAT=0)
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
<ipython-input-13-550ba18bf81d> in <module>
----> 1 xds.TEMP.xoak.sel(TLONG=220, TLAT=0)
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in sel(self, indexers, **indexers_kwargs)
246 """
247 if not getattr(self, '_index', False):
--> 248 raise ValueError(
249 'The index(es) has/have not been built yet. Call `.xoak.set_index()` first'
250 )
ValueError: The index(es) has/have not been built yet. Call `.xoak.set_index()` first
xds.xoak.sel(TLONG=220, TLAT=0)
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
<ipython-input-12-227cdaa281aa> in <module>
----> 1 xds.xoak.sel(TLONG=220, TLAT=0)
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in sel(self, indexers, **indexers_kwargs)
251
252 indexers = either_dict_or_kwargs(indexers, indexers_kwargs, 'xoak.sel')
--> 253 indices = self._query(indexers)
254
255 if not isinstance(indices, np.ndarray):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in _query(self, indexers)
135
136 def _query(self, indexers):
--> 137 X = coords_to_point_array([indexers[c] for c in self._index_coords])
138
139 if isinstance(X, np.ndarray) and isinstance(self._index, XoakIndexWrapper):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in coords_to_point_array(coords)
18
19 """
---> 20 c_chunks = [c.chunks for c in coords]
21
22 if any([chunks is None for chunks in c_chunks]):
~/miniconda3/envs/dcpy/lib/python3.8/site-packages/xoak/accessor.py in <listcomp>(.0)
18
19 """
---> 20 c_chunks = [c.chunks for c in coords]
21
22 if any([chunks is None for chunks in c_chunks]):
AttributeError: 'int' object has no attribute 'chunks'
%load_ext watermark
%watermark -d -iv -m -g
Compiler : GCC 9.3.0
OS : Linux
Release : 5.8.0-44-generic
Machine : x86_64
Processor : x86_64
CPU cores : 8
Architecture: 64bit
Git hash: bd1236ca615b32595c43cfa689e85fc9a112eb9f
numpy : 1.20.1
xoak : 0.1.0
xarray : 0.17.1.dev3+g48378c4b1
matplotlib: 3.3.4
pop_tools : 2020.12.15.post6+dirty